Find subarray with given sum
Input: arr[] = {1, 4, 20, 3, 10, 5}, sum = 33
Ouptut: Sum found between indexes 2 and 4
Input: arr[] = {10, 2, -2, -20, 10}, sum = -10
Ouptut: Sum found between indexes 0 to 3
Input: arr[] = {-10, 0, 2, -2, -20, 10}, sum = 20
Ouptut: No subarray with given sum exists
Naive Solution:
We pick elements one by one in outer loop and find its sum with other elements to its right till we get the given sum.
Code:
import java.util.*;
public class Solution {
public static void main(String[] args) {
int[] a = {10, 2, -2, -20, 10};
int n = a.length;
int sum = -10, currsum = 0;
int index1 = -1, index2 = -1;
for(int i=0; i<n; i++){
currsum = a[i];
for(int j = i+1; j<n; j++){
currsum +=a[j];
if(currsum == sum){
index1 = i;
index2 = j;
break;
}
}
if(currsum == sum){
break;
}
}
System.out.println(index1+" "+index2);
}
}
Output:
0 3
Efficient Solution: (with only non negative elements)
It is based on sliding window technique similar to https://www.geeksforgeeks.org/window-sliding-technique/
Code:
import java.util.*;
public class Solution {
public static void main(String[] args) {
int[] a = {1,4,20,3,10,5};
int n = a.length;
int sum = 33, currsum = a[0];
int index1 = 0, index2 = 0;
while(index2<n){
if(currsum < sum){
index2++;
currsum += a[index2];
}
if(currsum > sum){
currsum -= a[index1];
index1++;
}
if(currsum == sum){
break;
}
}
System.out.println(index1+" "+index2);
}
}
Output:
2 4
Efficient solution (negative numbers) : O(n) auxiliary space, O(n) time
Here we keep track of sum find so far in map. We will calculate the currsum. If currsum is equal to given sum we will print the indices. Else we will check if the difference between currsum and sum is present in the map. This can be better understood by the illustration following the code.
Code:
import java.util.*;
public class Solution {
public static void main(String[] args) {
int[] a = {1,4,-20,-3,-10,5};
int n = a.length;
int sum = -33, currsum = a[0];
Map<Integer,Integer> map= new HashMap<Integer,Integer>();
for(int i=0; i<n; i++){
currsum += a[i];
if(a[i] == currsum){
System.out.println("0"+" "+i);
break;
}
if(map.containsKey(currsum-sum)){
int index1 = map.get(currsum-sum)+1;
System.out.println(index1+" "+i);
break;
}
map.put(currsum,i);
}
}
}
Output:
2 4
Illustration:
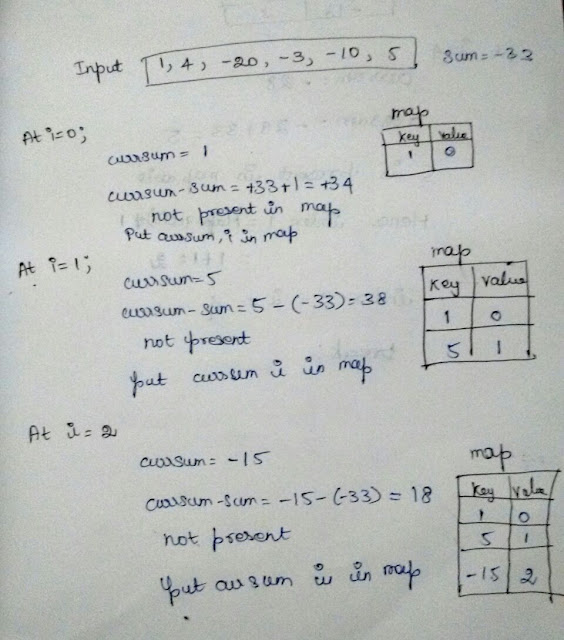

Comments
Post a Comment