Root to leaf path sum equal to a given number
Given a binary tree and a number, return true if the tree has a
root-to-leaf path such that adding up all the values along the path
equals the given number. Return false if no such path can be found.
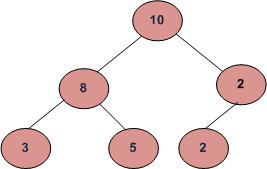
For example, in the above tree root to leaf paths exist with following sums.
21 –> 10 – 8 – 3
23 –> 10 – 8 – 5
14 –> 10 – 2 – 2
So the returned value should be true only for numbers 21, 23 and 14. For any other number, returned value should be false.
Code:
package trees;
public class N1906_SumRootToLeafPath {
Node root = null;
static class Node{
int data;
Node left, right;
Node(int data){
this.data = data;
left = right = null;
}
}
static boolean hasRootToLeafPath(Node node, int sum){
//if tree is called with null root
if(node == null){
return (sum == 0);
}
if(node.left == null && node.right == null){
return (sum == node.data);
}
int currsum = sum - node.data;
return hasRootToLeafPath(node.left, currsum) || hasRootToLeafPath(node.right, currsum);
}
public static void main(String[] args){
int sum = 14;
/* Constructed binary tree is
10
/ \
8 2
/ \ /
3 5 2
*/
N1906_SumRootToLeafPath tree = new N1906_SumRootToLeafPath();
tree.root = new Node(10);
tree.root.left = new Node(8);
tree.root.right = new Node(2);
tree.root.left.left = new Node(3);
tree.root.left.right = new Node(5);
tree.root.right.left = new Node(2);
System.out.println( hasRootToLeafPath(tree.root, sum));
}
}
Output:
true
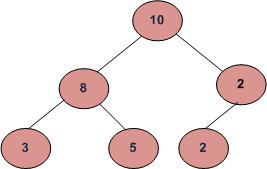
For example, in the above tree root to leaf paths exist with following sums.
21 –> 10 – 8 – 3
23 –> 10 – 8 – 5
14 –> 10 – 2 – 2
So the returned value should be true only for numbers 21, 23 and 14. For any other number, returned value should be false.
Code:
package trees;
public class N1906_SumRootToLeafPath {
Node root = null;
static class Node{
int data;
Node left, right;
Node(int data){
this.data = data;
left = right = null;
}
}
static boolean hasRootToLeafPath(Node node, int sum){
//if tree is called with null root
if(node == null){
return (sum == 0);
}
if(node.left == null && node.right == null){
return (sum == node.data);
}
int currsum = sum - node.data;
return hasRootToLeafPath(node.left, currsum) || hasRootToLeafPath(node.right, currsum);
}
public static void main(String[] args){
int sum = 14;
/* Constructed binary tree is
10
/ \
8 2
/ \ /
3 5 2
*/
N1906_SumRootToLeafPath tree = new N1906_SumRootToLeafPath();
tree.root = new Node(10);
tree.root.left = new Node(8);
tree.root.right = new Node(2);
tree.root.left.left = new Node(3);
tree.root.left.right = new Node(5);
tree.root.right.left = new Node(2);
System.out.println( hasRootToLeafPath(tree.root, sum));
}
}
Output:
true
Comments
Post a Comment